未发布 界面控件Essential Studio for ASP.NET Web Forms 发布2017 v4丨附下载 新的控件
组合框组件允许用户输入一个值或从预定义选项列表中选择一个选项。单击此组件的箭头图标时,下拉列表将显示用户可以从中选择的值列表。
主要特征
数据绑定:允许绑定和访问本地或服务器端数据源的项目列表。
分组:支持将单个或特定类别下的逻辑相关项目进行分组。
排序:支持按字母顺序(升序或降序)排序列表项。
过滤:允许根据组件中输入的字符过滤列表项目。
模板:允许自定义列表项目、选定的值、页眉、页脚、类别组页眉。
可访问性:内置的可访问性支持有助于通过键盘、屏幕阅读器或其他辅助技术设备访问所有组件的功能。
拼写检查器
新的控件
新的拼写检查器控件通过将它们与存储的字典单词列表进行比较,查找并突出显示拼写错误的单词。
主要特征
AUTOCOMPLETE
搜索索引多栏弹出
现在可以区分多列AUTOCOMPLETE控件中的显示格式和搜索字段,允许用户在列表中搜索任意数量的字段,而无需修改所选的文本格式。
CHART
轴标签
支持显示多行轴标签。
显示外部数据标签
现在可以在图表区域内显示部分可见的数据标签。
数据管理器
防伪
防伪令牌可用于帮助保护应用程序免受跨站请求伪造。它生成一个隐藏的表单字段(防伪造标记),在提交表单时进行验证。
额外的数据操作
提供了IN和NotIN过滤器操作用于在WHERE过滤器中指定多个值。
DIAGRAM
对齐
支持定制用户手柄的位置。
端口增强
现在可以拖动端口来重新定位端口。
对称布局
对称布局是用于以圆形和对称方式排列图的自动布局。
标签交互
已经为标签提供了交互支持。他们可以被选中、拖动、调整大小和旋转。
DOCIO
增强Word转换成PDF功能
DocIO现在允许将带有行号的Word文档以其原始格式转换为PDF。
下拉列表
服务器过滤
在搜索框中输入文本时,此功能将过滤数据源。过滤是基于包含来自整个数据源的匹配项。
服务器过滤:远程数据
服务器过滤:本地数据
文件管理器
支持鼠标选择
支持在文件资源管理器控件中使用鼠标管理文件/文件夹。
甘特图
资源分配视图
甘特图控件支持资源分配视图。使用该视图,用户可以解释在同一日期发生的任务以及项目中资源的过度分配。
排序
现在可以根据行顺序显示具有序列号的任务。
多行选择
现在可以选择多行并执行缩进和负缩进操作。
列过滤
现在可以过滤甘特图控件中的特定字段或列。
展开/折叠记录
甘特图控制现在支持扩展和折叠特定级别的记录。
表格
在Excel过滤器菜单中添加当前选项进行过滤
在搜索时显示添加当前选择到过滤器复选框,允许用户在表格中添加当前选中或清除的复选框列表数据以及已经过滤的数据。
导出模板列
使用Excel、PDF和Word文件格式导出包含模板列的表格。
导出详细信息模板
导出使用Excel、PDF和Word文件格式的详细信息模板。
KANBAN
外部拖放
KANBAN控件现在支持与其他控件之间的拖放操作。
没有数据源的KANBAN列
KANBAN列可以在不绑定数据源的情况下显示。
PIVOT客户端
支持Mondrian服务器(客户端模式)
分页将大记录拆分为不同的段,便于查看数据。它还改善了连接到Mondrian服务器的PIVOT控件的性能。
PIVOT网格
支持Mondrian服务器(客户端模式)
分页将大记录拆分为不同的段,便于查看数据。它还改善了连接到Mondrian服务器的PIVOT控件的性能。
RIBBON
设计器
改进了ASP.NET Web Forms中功能区组件的设计器。
滑块
添加按钮
添加了一个可以隐藏的按钮,允许增加和减少滑块的值。
ESSENTIAL XLSIO
图表转换为图像
图表元素(如图例,标题,绘图区和显示单元)的手动布局位置现在可以正确转换为图像。
未发布 CAD .NET v12发布,提高GDI+可视化速度并可导入AutoCAD®DWG 2018丨附下载
CAD .NET新版本大大提高了GDI+可视化的速度。当加载具有复杂结构的大文件时可以很明确的体验到。例如,包含大量实体的一些文件的可视化速度现在提升了十倍。
更重要的是,CAD .NET现在可以导入最近发布的最新DWG版本的AutoCAD®DWG 2018。
此外,CAD .NET使用非Unicode SHX文本和形状的方式已经大大改善。这种改进对于使用本地非Unicode字体的系统尤其重要,例如德语、法语、希伯来语和其他语言的特殊字符。
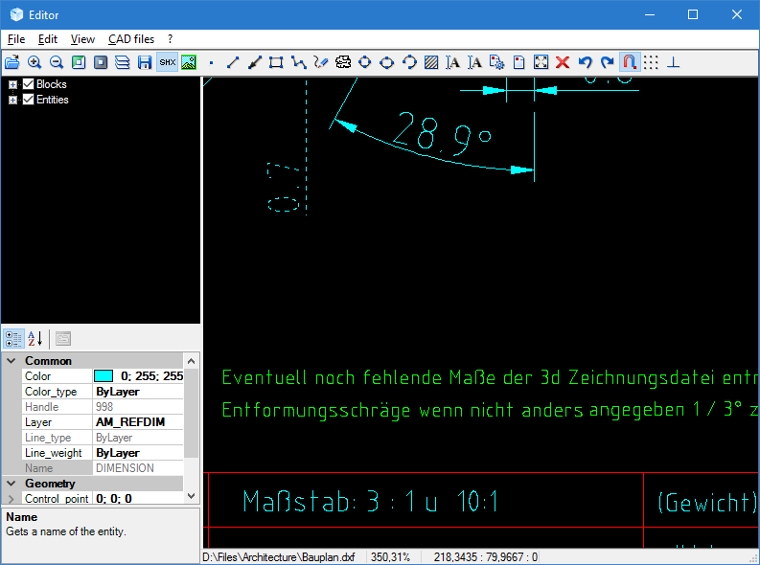
CAD .NET 12包含的改进和修复的内容列表:
GDI+可视化速度大大提高
导入AutoCAD®DWG 2018
改进非Unicode SHX文本/形状支持
Bug修复
试用、下载、了解更多产品信息请点击"咨询在线客服"
未发布 案例实战:LightningChart集成Dynamotive汽车遥测软件|见证真正无与伦比的性能 案例研究日期:2017年3月
经营行业:汽车工程
成立:1998
LightningChart解决方案:Various
高性能的控制系统
Dynamotive是ABB集团的一员,是一个主要部署在汽车行业的高性能实时控制系统的高档品牌供应商。Dynamotive为主要汽车制造商及其遍布欧洲、美国和亚洲的供应商提供测试设备和工具软件。
LightningChart®提供真正无与伦比的性能
“Dynamotive在对众多的选择进行鉴定后选择了LightningChart。没有任何一种替代方案能够做到在性能方面如此接近完美。”
——软件开发经理安德鲁·普尔(Andrew Poole)
Dynamotive使用LightningChart从控制系统实时提供数据。他们在LightningChart周围建立了一个解决方案,可以同时并且持续地以多个采样率显示和记录数据。采样率从1Hz到50 kHz不等,屏幕刷新率可以调节高达50 Hz,为用户提供了关于控制过程决策所需的信息。
当被问及LightningChart的最佳优势时,Poole先生给出答复:“LightningChart的最佳功能是性能,性能和性能。”
示例:表示运行节气门循环的图像
快速和容易实现
LightningChart给予Dynamotive的主要优点是使他们能够快速开发可靠和高性能的实时数据。“除了使用LightningChart为我们的控制系统实现高性能实时数据趋势,我们可以在两周内构建一个驱动程序辅助应用程序(使车辆的驾驶员能够遵循道路简档),早期的实施需要几个月 需要尝试并获得体面的图形性能,但与LightningChart的优异性能是给定的。”软件开发经理Poole如是介绍。
Dynamotive团队并没有真的必须使用技术支持。 安德鲁·普尔(Andrew Poole):“我们是LightningChart的早期使用者,当时有一些来自Arction创始人的电子邮件让我们犹豫了,但之后,我们需要做的大部分工作都很容易实现,这是因为拥有了直接和结构合理的API。”
观看视频,了解LightningChart如何整合到Dynamotive开发的汽车遥测软件中:
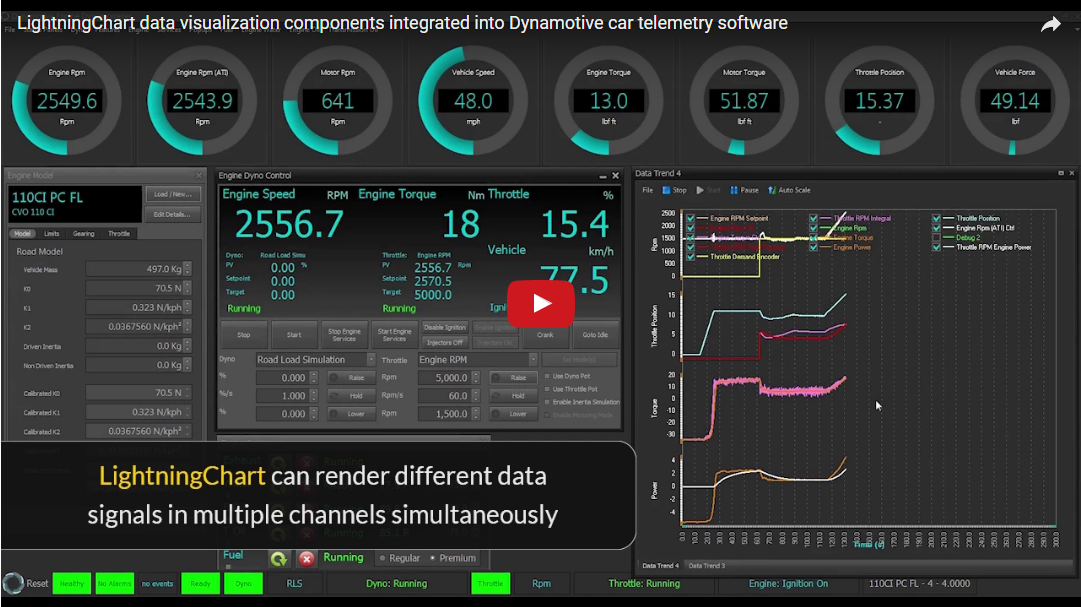
未发布 5个优秀编码挑战帮你训练大脑,你敢尝试吗? 每个人都知道编程正在成为几乎每个行业的重要组成部分,它对组织的帮助和对大型系统的维护是独一无二的,因此越来越多的人开始了他们的编程旅程。你可以从你觉得合适和方便的任何交互式平台和书籍中学习编程。但是这还不够,我们应该练习更多新的东西。
编码与你的创造力、创新能力密切相关。但很多时候,我们花费大量时间来处理常见问题而忘记了创造力。我不太确定这是否是编码挑战出现的原因,但它们肯定可以帮助你去思考。
我们可以说编程挑战是伟大的:
学习新的做事方式
练习一种新的编程语言
解决遇到的关键问题
保持我们的大脑活跃和集中力
以及玩得开心!
在寻找最好的编程挑战过程中筛选了五个非常好的资源。相信这对你的编程旅途有所帮助,并探索更大的计算机科学领域。
未发布 VectorDraw Developer Framework v7.7012.0.4发布丨附下载
VectorDraw Developer Framework(VDF)v7.7012.0.2更新内容:
WebJS
新增需求(7.7012.0.3)
漏洞(7.7012.0.2)
漏洞(7.7012.0.3)
Engine
漏洞(7.7012.0.2)
70001114 手柄选择未正确更新,并且屏幕上仍然显示
70001116 UpdatePropertiesFromPrinter丢失纸张信息
70001117 GetLineTypeDlg的Wrapper不会返回正确的行类型
漏洞(7.7012.0.3)
未发布 JMS消息平台FioranoMQ更新v10.3.0,改进共享HA功能 FioranoMQ是世界上第一个基于网格、点对点JMS消息平台,具有强大且独特的功能,包括动态路由、消息流的分布式调试、JMS客户端应用程序的动态部署、无限的可扩展性、直接援引标准的JCA组件以及无与伦比的JMX标准易管理功能。在封闭的基准测试中超过每秒50,000条的消息,FioranoMQ还是世界上最快的独立的标准消息服务器。
v10.3.0新增
改进FioranoMQ共享HA(高可用性)功能
共享HA功能已得到增强,使其对活动代理和共享数据库以及锁定文件之间的网络中断更敏感。通过检查锁定文件是通过NFS共享创建还是使用本地文件,增加了更多安全检测来检查锁定文件上代理的真实性。
新增的共享HA代理解决了以下问题:
当活动代理和共享数据库之间的网络连接断开时更改状态。
数据库和锁定文件运行状况检查:如果活动代理与共享数据库的断开连接,代理将自动进行故障切换。
HA状态线程不会在任何情况下退出。
切换到被动状态后,活动代理必须释放锁,让待机代理获取锁并变为活动状态。
共享HA部署必须满足以下条件:
CSP磁盘使用通知
当启用生产者故障快速功能时,“客户端持久性”(CSP)的磁盘使用率可能非常频繁,具体取决于将消息发送到队列或主题目标时设置的超时值。因此,引入了CSP磁盘使用警报机制。客户端应用程序通过在客户端进行日志记录并在特定生产应用程序的CSP的使用违反配置的阈值时发出提醒。
注意:此功能现在可在Java和C/C++ RTL中使用。
优化加载管理对象
在代理启动期间或通过JMX API调用时,通过XML文件加载管理对象已被优化,以处理更多数量的目标配置。优化确保使用某些常用目的地的客户端应用程序不会受到影响,从而避免长时间停顿。
MQTT安全和认证
支持创建与MQTT代理程序的安全连接。
CSharp RTL(JMS 2.0)的异步发送功能
新的发送方法已添加到MessageProducer中,允许消息异步发送。这些方法立即返回并在单独的线程中执行发送,而不阻塞调用线程。
JMX API
添加以下JMX API:
Connection MBean中的API来获取客户端版本信息和构建号。
主题会话MBean下的API'getPersistentQueueSize()'和'getPSQAvailableMessageCount()'。
'listAllSessionsInfo()',所有主题会话的列表及其PSQ度量。
'getClientID(TopicSessionToken)'获取与主题会话令牌相关联的客户机ID。
'get(set)LockFileValidationTimeout()',在共享HA中锁定文件验证超时。
资源管理器mbean下的'getResourceCount()'分别获取由资源名称和实例计数组成的地图作为关键值。
CSharp RTL
在CSharp RTL中添加了新的管理API,用于限制基于IP地址的FioranoMQ代理。
“未接来电”,当代理人关机时调用的方法将在代理程序启动时存储和执行。此功能现在可在C#RTL中使用。
改变
FioranoMQ 10.3.0代理和Java RTL需要Java 8或更高版本。
基于net beans的Studio工具将不再与FioranoMQ安装程序一起发送,与安装程序一起提供的基于eclipse的Studio将针对64位平台。
共享HA条件:
HA对(Primary-Secondary)通信和网关服务器通信必须共享相同的网络接口卡。
锁定文件和共享数据库必须共享相同的装载点。
HA中的代理不得与HAManager属性“Primary”共享相同的值。
最新的C/C++ RTL库现在使用VS 2015构建。
未发布 Windows网络守门人UserLock教程:UserLock教程:阻止规定时间以外的访问连接 UserLock免费下载试用>>>
UserLock允许您创建规则用来阻止用户在授权时间以外连接到网络。本文将一步一步的教您如何制定规则来阻止用户工作站和终端连接超出工作时间:
- 周一至周五,8:00至19:00。
- 周六上午,9:00至13:00。
并且将在到达时限后强制任何会话的注销打开。
1、点击菜单中的“受保护的帐户”。如果已存在则可以通过双击相应的行来打开所需的用户帐户。否则,您可以为目标组创建受保护的帐户。
2、显示“时间限制”部分。
3、选择“以下时间范围”下拉列表的'被授权'选项。
4、点击“添加”来定义时间段。
5、定义具体的时间范围。选择“交互”作为会话类型来选择哪些类型的会话,包括工作站和终端会话类型。点击“确定”。
6、检查相应的日期框 - 星期一至星期五。点击“确定”。
7、调整时间为上午8:00至下午7:00。点击“确定”以验证第一个授权的时间范围。
8、您现在必须为星期六定义第二个时间范围。再次点击“添加”。
9、定义第二个时间框架,即星期六上午9:00至下午1:00。再次选择“交互”作为会话类型,包括工作站和终端会话类型。点击“确定”。
10、取消选中从星期一到星期五的框,而是选中星期六的框。点击“确定”。
11、调整时间为上午9:00至下午1:00。点击“确定”添加第二个时间框架。
您已经成功定义了与授权时间相对应的两个时间范围。用户在这些时间以外的连接将被拒绝。
12、要在时间限制后注销用户会话,请调整“超时时采取的行动”为“注销会话”选项。
请注意,强制注销表示所有未保存的文档将丢失。下一步允许您自定义警。
13、要警告用户授权时间已经结束,将“注销通知超时”设置为“已启用”,并输入几分钟,在此分钟内将显示警告消息框(在我们的示例中为15)。
14、在“快速访问”面板中单击“确定”以验证规则。
“Everyone”组的用户成员将被允许在授权时间内打开工作站或终端会话的权限。达到时限的会话将被注销,并发出预警通知。所有工作站和终端连接超出规定的时间将被拒绝。
您可以在“消息”视图中自定义“注销通知超时”内容。通知消息参考是“TIME_RESTRICTION_LOGOFF”。
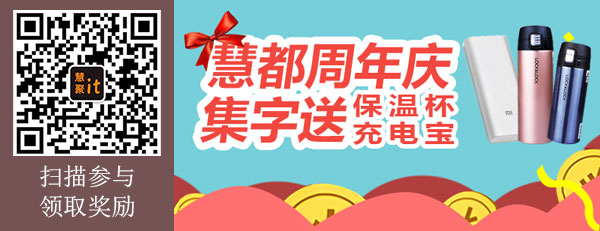